Textblob is what you want for a quick and easy sentiment analysis
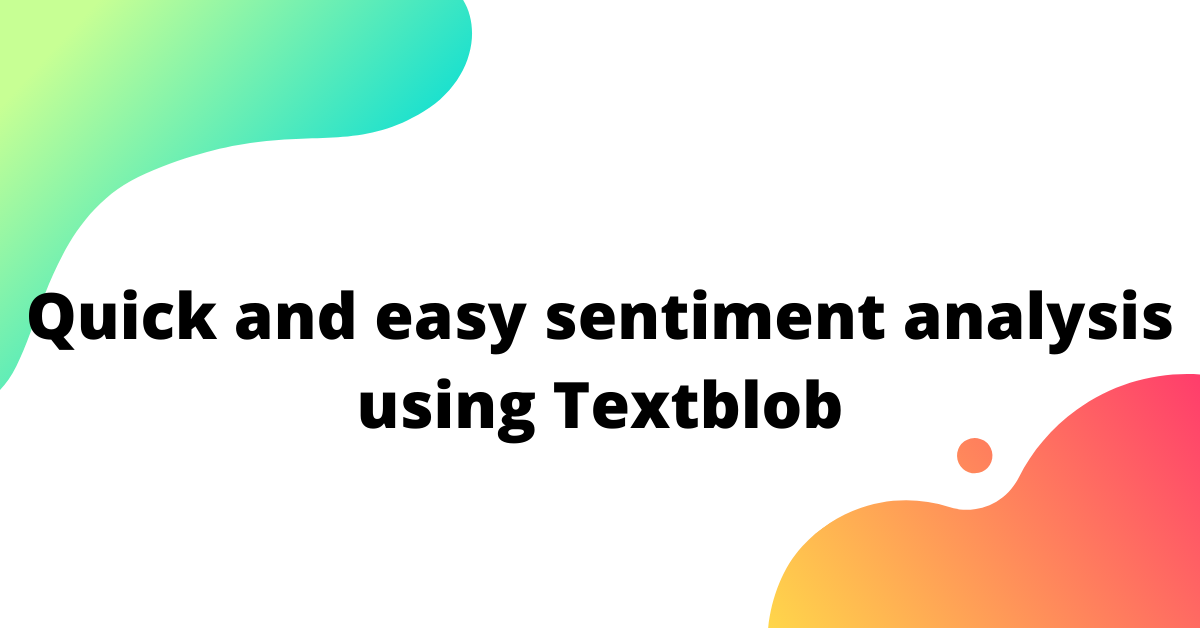
In the context of an analysis of text data, sentiment analyses are often a suitable means of generating additional insights from texts. When it comes to texts in English, Textblob is a great way to achieve good results with little time. If you want to analyse German texts, for example, sentiws is a good alternative that is similarly quick and easy to use.
In this post I show you how you can use Textblob yourself to do sentiment analysis on your text data.
First, make sure you have the latest version of Python 3 installed. Then you can run the following code in your shell to install Textblob. You can use pip or conda for the installation. The pip command is:
pip install -U textblob
python -m textblob.download_corpora
If you want to use conda, make sure to create or activate your environment and run:
conda install -c conda-forge textblob
python -m textblob.download_corpora
Get started with Textblob
Now, create a python script and you are ready to start. You can, for example, play around with Textblob a bit and run the following code to create a first blob object and have the part-of-speech tags or the sentiment polarity as output.
from textblob import TextBlob
text = TextBlob('This is a good example.')
print(text.tags)
print(text.sentiment.polarity)
The result of the sentiment polarity lies between [-1,1]. The closer the value is at -1 the more negative the sentiment is - the closer it is to 1 the more positive. In general, positive values imply a positive sentiment while negative values imply a negative sentiment.
Create your Textblob script to analyse large amounts of text data and save results
I assume you are reading this article because you have a dataset with lots of text data for which you have sentiment scores. Therefore, I will now show you how you can generate the desired output.
Before you start you need to install pandas. Please run the following in your shell:
conda install pandas
Now we can start modifying our script. To start with, make sure to not only import Textblob but also import pandas.
import pandas as pd
from textblob import TextBlob
Then you load your .csv file that contains a column with the text data that should be analyzed.
df = pd.read_csv('your_file_name.csv', sep=',')
text = df['The_column_where_your_text_is_in']
Then you create empty arrays to store temporary and final results in:
sentiment = []
sentiment_temp = []
result = []
Then we loop over every text, perform the analysis and save results. But before that we define a function that calculates the average of something.
def Average(lst):
return sum(lst) / len(lst)
for elem in text:
blob = TextBlob(elem)
for sentence in blob.sentences:
sentiment_temp.append(sentence.sentiment.polarity)
sentiment = Average(sentiment_temp)
result.append(sentiment)
df['Sentiment'] = result
Finally you save the resulting data frame as .csv file so that you can use the results for further analyses.
df.to_csv("./new_file_name.csv", sep=',', index=False)
You should now have your initial data set, enriched with a sentiment score per observation. If you want to increase the accuracy of the sentiment analysis, you could use the Naive Bayes Analyzer, for example.
As always, you can find the complete code on Github.